User
In our API, a User serves as the primary entity and can possess multiple Profiles to represent different contexts or settings. Specifically, a User can have one personal Profile and multiple business Profiles. Each Profile - whether personal or business - can have its own multi-currency account, enabling transactions across various currencies. This hierarchical structure allows for flexible management of user settings and financial operations, accommodating both personal and business needs.
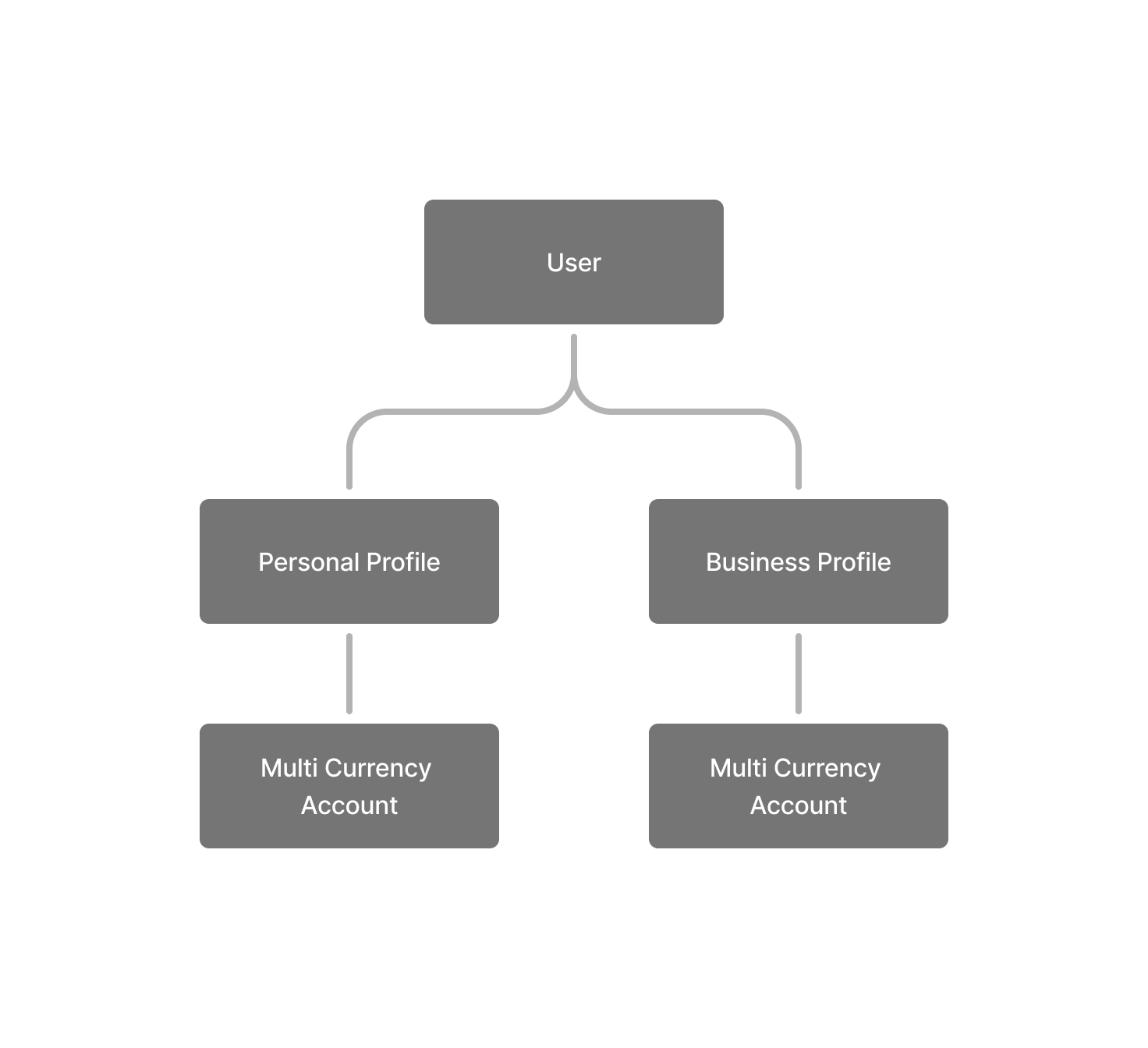
userId
User's full name
User's email
If user is active or not
User's first name
User's lastname
Phone number
Date of birth
Person's occupation
Link to person avatar image.
Address object ID to use in addresses endpoints
Address country code in 2 digits. "US" for example
Address first line
Address post code
Address city name
Address state code State code. Required if country is US, CA, AU, BR.
User occupation. Required for US, CA, JP
{"id": 101,"name": "Example Person","email": "person@example.com","active": true,"details": {"firstName": "Example","lastName": "Person","phoneNumber": "+37111111111","occupation": "","address": {"city": "Tallinn","countryCode": "EE","postCode": "11111","state": "","firstLine": "Road 123"},"dateOfBirth": "1977-01-01","avatar": "https://lh6.googleusercontent.com/photo.jpg","primaryAddress": 111}}
GET /v1/me
Get authenticated user details for the user's token submitted in the Authorization header. Response includes also personal user's profile info.
Response
Returns a user object
curl -X GET https://api.sandbox.transferwise.tech/v1/me \-H 'Authorization: Bearer <your api token>'
GET /v1/users/{{userId}}
Get authenticated user details by user ID. Response includes also personal user's profile info.
Response
Returns a user object
curl -X GET https://api.sandbox.transferwise.tech/v1/users/{{userId}} \-H 'Authorization: Bearer <your api token>'
POST /v1/user/signup/registration_code
Wise uses email address as unique identifier for users. If email is new (there is no active user already) then new user will be created.
When you are submitting an email which already exists amongst our users then you will get a warning that "You’re already a member. Please login". If user already exists then you need to redirect to "Get user authorization" webpage.
New user's email address
Randomly generated registration code that is unique to this user and request. At least 32 characters long. You need to store registration code to obtain access token on behalf of this newly created user in next step. Please apply the same security standards to handling registration code as if it was a password.
User default language for UI and email communication. Allowed values EN, US, PT, ES, FR, DE, IT, JA, RU, PL, HU, TR, RO, NL, HK. Default value EN.
Response
userId
User full name. Empty.
Customer email
true
User details. Empty.
curl -X POST https://api.sandbox.transferwise.tech/v1/user/signup/registration_code \-H 'Authorization: Bearer <client credentials token>' \-H 'Content-Type: application/json' \-d '{"email": "user@email.com","registrationCode": <unique guid for the user>,"language": "EN"}'
{"id": 12345,"name": null,"email": "new.user@domain.com","active": true,"details": null}
{"errors": [{"code": "NOT_UNIQUE","message": "You’re already a member. Please login","path": "email","arguments": ["email","class com.transferwise.fx.api.ApiRegisterCommand","existing.user@domain.com"]}]}
POST /v1/users/exists
Wise uses email address as unique identifier for users. If email has already been used by a user, it cannot be reused to create a new user.
Note that this uses a client-credentials-token
and not a user access_token
for authentication.
User's email address
Response
Email has already exist
curl -X POST https://api.sandbox.transferwise.tech/v1/users/exists \-H 'Authorization: Bearer <client credentials token>' \-H 'Content-Type: application/json' \-d '{"email": "test@wise.com"}'
{"exists": true}
PUT /v1/users/{{userId}}/contact-email
Sets a contact email address. The contact email address is used to send notifications to users who have been registered with a dummy email address.
Contact email address
Response
Returns a contact email object.
Contact email address
curl -X PUT https://api.sandbox.transferwise.tech/v1/users/{{userId}}/contact-email \-H 'Authorization: Bearer <your api token>' \-H 'Content-Type: application/json' \-d '{"email": "new-user@email.com"}'
{"email": "new-user@email.com"}
GET /v1/users/{{userId}}/contact-email
Retrieves a contact email address.
Response
Returns a contact email object.
Contact email address
curl -X GET https://api.sandbox.transferwise.tech/v1/users/{{userId}}/contact-email \-H 'Authorization: Bearer <your api token>'
{"email": "new-user@email.com"}